In Python, comparison operators are used to evaluate the relationship between two values. They return either True
or False
based on whether the condition being checked holds true or not. These operators are key when you need to perform conditional logic, like comparing if one number is larger than another or checking equality between two values.
But before this we should know about the Python
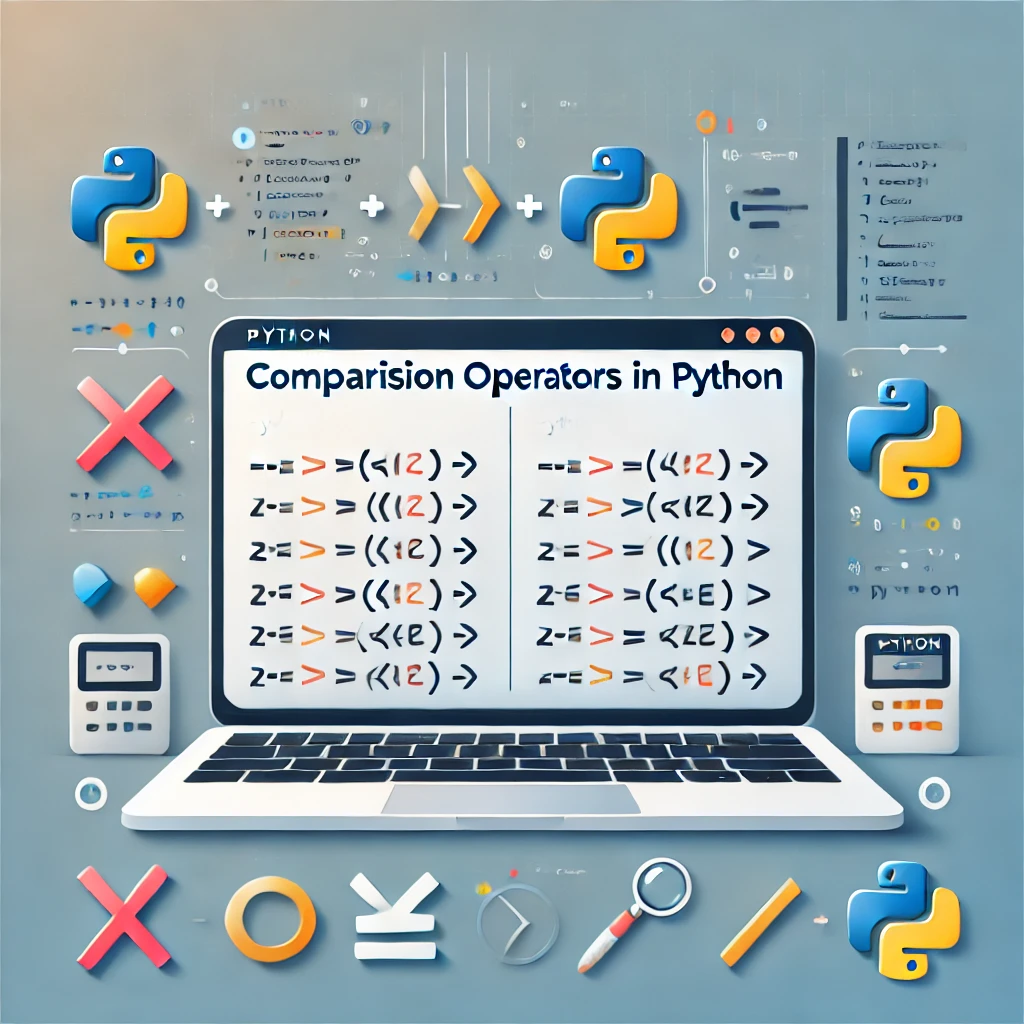
Operator | Description | Example | `Result |
== | Equal to | x == y | True if x and y are equal |
!= | Not equal to | x != y | True if x and y are not equal |
> | Greater than | x > y | True if x is greater than y |
< | Less than | x < y | True if x is less than y |
>= | Greater than or equal to | x >= y | True if x is greater than or equal to y |
<= | Less than or equal to | x <= y | True if x is less than or equal to y |
These operators are used to compare two values or variables and return True or False based on the comparison result. |
Common Comparison Operators in Python
- Equal to (
==
)
This operator checks if two values are equal.
Returns True
if the values are the same, otherwise False
.
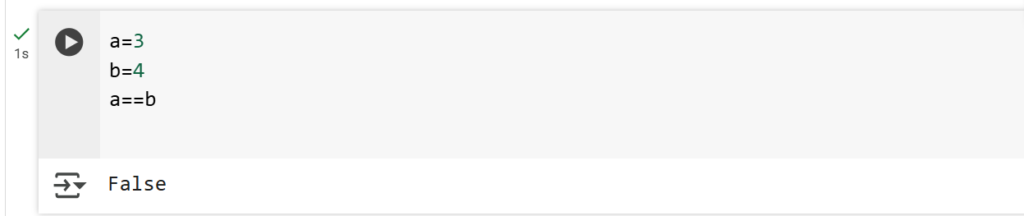
2. Not equal to (!=
)
Returns True
if the values are different, otherwise False
.
This operator checks and confirms if the values of both are not equal.
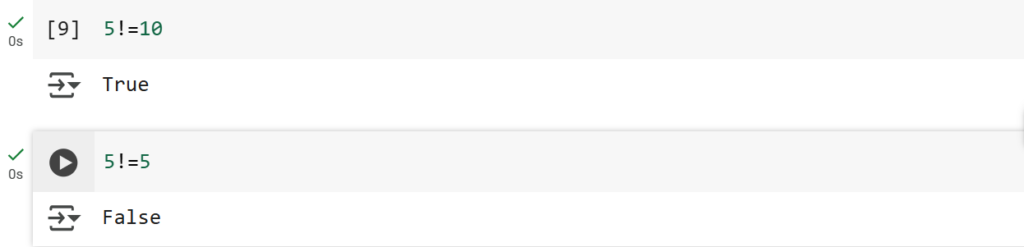
3.Greater than (>
)
Returns True
if the left value is greater, otherwise False
.
This operator checks if the value shown on the left side is greater than the right value.
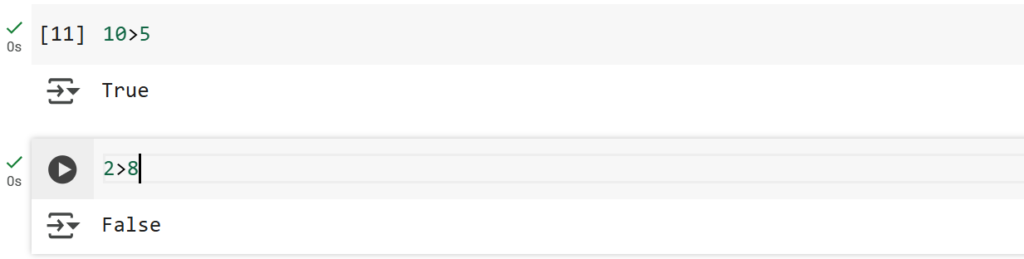
4.Less than (<
)
Returns True
if the left value is less, otherwise False
.
This operator checks if the value shown on the left side is less than the right value.
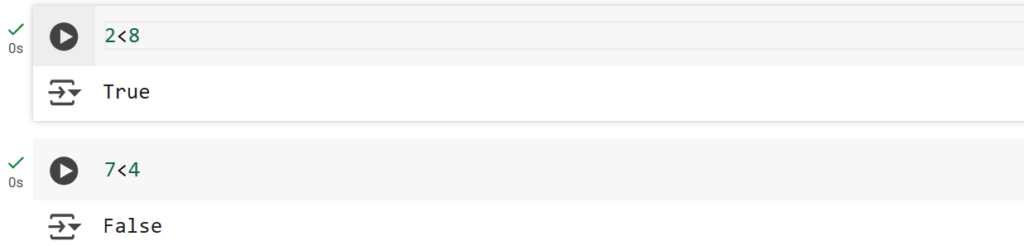
5. Greater than or equal to (>=
)
This Returns True
if the value shown on the left is greater or equal, otherwise False
.
This operator checks if the left value is greater than or equal to the right value.
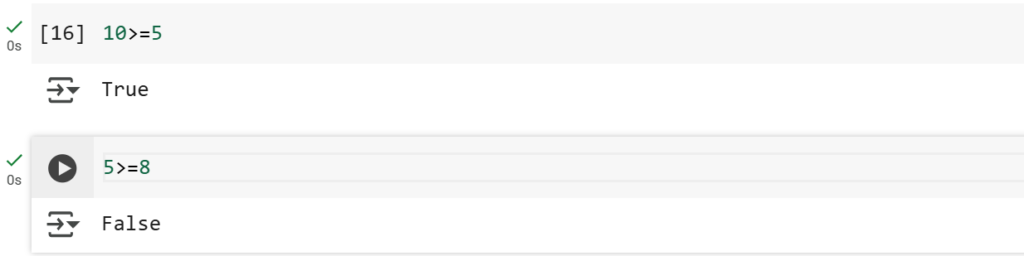
6. Less than or equal to (<=
)
This operator checks if the left value is less than or equal to the right value. Returns True
if the left value is less or equal, otherwise False
.
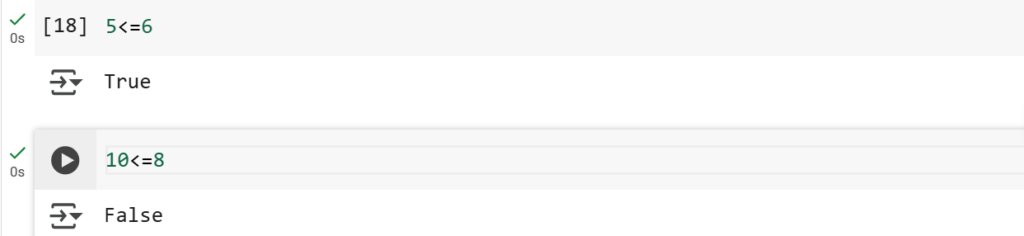
Conclusion
Comparison operators in Python are fundamental tools for making decisions and controlling the flow of your program. Whether you’re checking equality, testing greater-than or less-than conditions, or combining multiple comparisons, these operators are essential in any Python developer’s toolkit.
By understanding and applying comparison operators, you’ll be able to write more dynamic and efficient Python code that reacts to different inputs and conditions.
Jobs available for PYTHON